Primitives
Abstract
#
Meshes.Primitive
— Type
Primitive{M,CRS}
We say that a geometry is a primitive when it can be expressed as a single entity with no parts (a.k.a. atomic). For example, a sphere is a primitive described in terms of a mathematical expression involving a metric and a radius. See https://en.wikipedia.org/wiki/Geometric_primitive.
Concrete
Point
#
Meshes.Point
— Type
Point(x₁, x₂, ..., xₙ)
Point((x₁, x₂, ..., xₙ))
A point in Dim
-dimensional space with coordinates in length units (default to meters).
The coordinates of the point are given with respect to the canonical Euclidean basis, and integer coordinates are converted to float.
Examples
# 2D points
Point(1.0, 2.0) # add default units
Point(1.0m, 2.0m) # double precision as expected
Point(1f0km, 2f0km) # single precision as expected
Point(1m, 2m) # integer is converted to float by design
# 3D points
Point(1.0, 2.0, 3.0) # add default units
Point(1.0m, 2.0m, 3.0m) # double precision as expected
Point(1f0km, 2f0km, 3f0km) # single precision as expected
Point(1m, 2m, 3m) # integer is converted to float by design
Notes
-
Integer coordinates are not supported because most geometric processing algorithms assume a continuous space. The conversion to float avoids
InexactError
and other unexpected results.
rand(Point, 100) |> viz
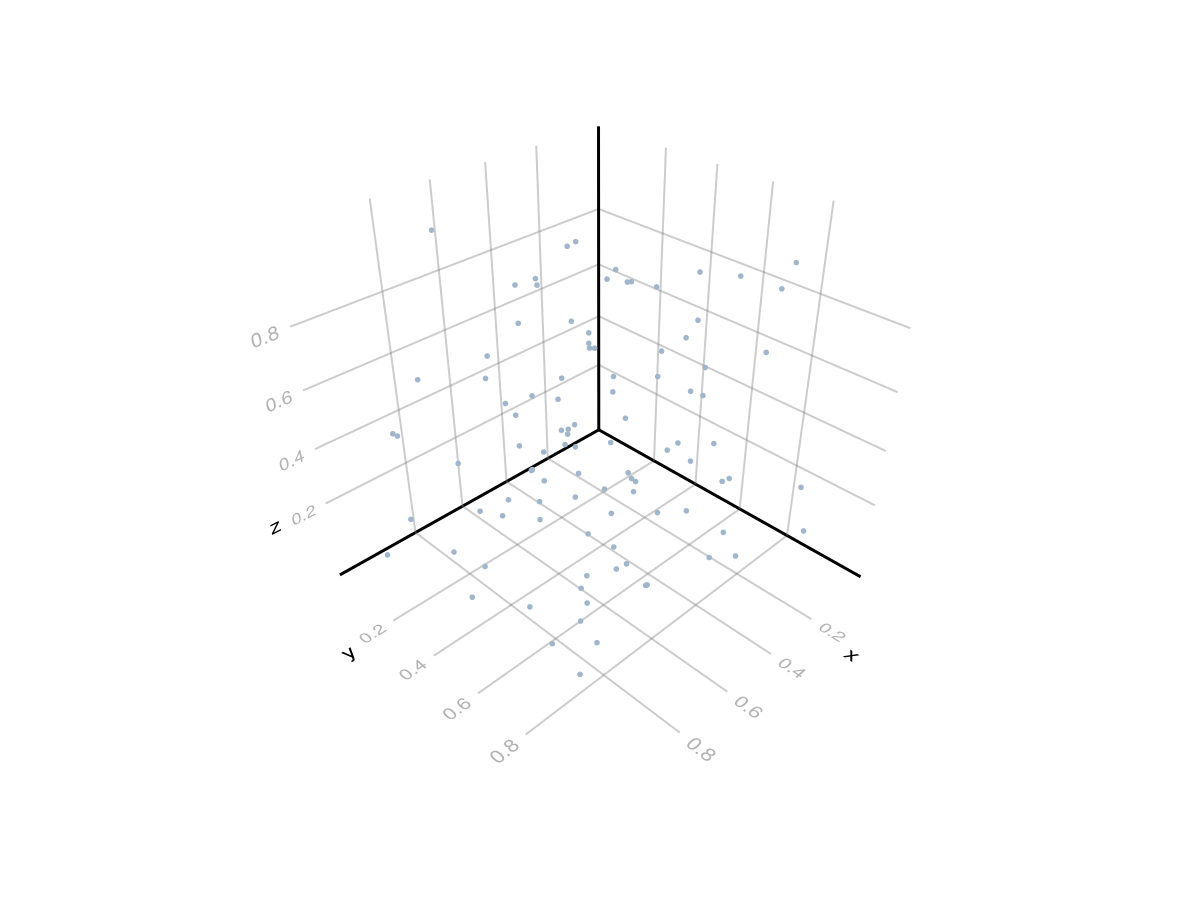
#
Base.:+
— Method
+(A::Point, v::Vec)
+(v::Vec, A::Point)
Return the point at the end of the vector v
placed at a reference (or start) point A
.
#
Base.:-
— Method
-(A::Point, v::Vec)
-(v::Vec, A::Point)
Return the point at the end of the vector -v
placed at a reference (or start) point A
.
Ray
#
Meshes.Ray
— Type
Ray(p, v)
A ray originating at point p
, pointed in direction v
. It can be called as r(t)
with t > 0
to cast it at p + t * v
.
BezierCurve
#
Meshes.BezierCurve
— Type
BezierCurve(points)
A recursive Bézier curve with control points points
. See https://en.wikipedia.org/wiki/Bézier_curve. A point on the curve b
can be evaluated by calling b(t)
with t
between 0
and 1
. The evaluation method defaults to DeCasteljau’s algorithm for accurate evaluation. Horner’s method, faster with a large number of points but less precise, can be used via b(t, Horner())
.
Examples
BezierCurve([(0.,0.),(1.,-1.)])
BezierCurve((0.,0.), (1.,0.), (1.,1.)) |> viz
ParametrizedCurve
#
Meshes.ParametrizedCurve
— Type
ParametrizedCurve(fun, range = (0.0, 1.0))
A parametrized curve is a curve defined by a function fun
that maps a (unitless) parameter t
in the given range
to a Point
in space.
Examples
ParametrizedCurve(t -> Point(cos(t), sin(t)), (0, 2π))
ParametrizedCurve(t -> Point(cos(t), sin(t), 0.2t), (0, 4π)) |> viz
Plane
#
Meshes.Plane
— Type
Plane(p, u, v)
A plane embedded in R³ passing through point p
, defined by non-parallel vectors u
and v
.
Plane(p, n)
Alternatively specify point p
and a given normal vector n
to the plane.
Box
#
Meshes.Box
— Type
Box(min, max)
A (geodesic) box with min
and max
points on a given manifold.
Examples
Construct a 3D box using points with Cartesian coordinates:
Box((0, 0, 0), (1, 1, 1))
Likewise, construct a 2D box on the plane:
Box((0, 0), (1, 1))
Construct a geodesic box on the ellipsoid:
Box(Point(LatLon(0, 0)), Point(LatLon(1, 1)))
Box((0.,0.,0.), (1.,1.,1.)) |> viz
Ellipsoid
#
Meshes.Ellipsoid
— Type
Ellipsoid(radii, center=(0, 0, 0), rotation=I)
A 3D ellipsoid with given radii
, center
and rotation
.
Ellipsoid((3., 2., 1.)) |> viz
Cylinder/CylinderSurface
#
Meshes.Cylinder
— Type
Cylinder(bottom, top, radius)
A solid circular cylinder embedded in R³ with given radius
, delimited by bottom
and top
planes.
Cylinder(start, finish, radius)
Alternatively, construct a right circular cylinder with given radius
along the segment with start
and finish
end points.
Cylinder(start, finish)
Or construct a right circular cylinder with unit radius along the segment with start
and finish
end points.
Cylinder(radius)
Finally, construct a right vertical circular cylinder with given radius
.
#
Meshes.CylinderSurface
— Type
CylinderSurface(bottom, top, radius)
A circular cylinder surface embedded in R³ with given radius
, delimited by bottom
and top
planes.
CylinderSurface(start, finish, radius)
Alternatively, construct a right circular cylinder surface with given radius
along the segment with start
and finish
end points.
CylinderSurface(start, finish)
Or construct a right circular cylinder surface with unit radius along the segment with start
and finish
end points.
CylinderSurface(radius)
Finally, construct a right vertical circular cylinder surface with given radius
.
Cylinder(1.0) |> viz
Cone/ConeSurface
#
Meshes.Cone
— Type
Cone(base, apex)
A cone with base
disk and apex
. See https://en.wikipedia.org/wiki/Cone.
See also ConeSurface
.
#
Meshes.ConeSurface
— Type
ConeSurface(base, apex)
A cone surface with base
disk and apex
. See https://en.wikipedia.org/wiki/Cone.
See also Cone
.
Cone(Disk(Plane((0,0,0), (0,0,1)), 1), (0,0,1)) |> viz
Frustum/FrustumSurface
#
Meshes.Frustum
— Type
Frustum(bot, top)
A frustum (truncated cone) with bot
and top
disks. See https://en.wikipedia.org/wiki/Frustum.
See also FrustumSurface
.
#
Meshes.FrustumSurface
— Type
FrustumSurface(bot, top)
A frustum (truncated cone) surface with bot
and top
disks. See https://en.wikipedia.org/wiki/Frustum.
See also Frustum
.
Frustum(
Disk(Plane((0,0,0), (0,0,1)), 2),
Disk(Plane((0,0,10), (0,0,1)), 1)
) |> viz
Torus
#
Meshes.Torus
— Type
Torus(center, direction, major, minor)
A torus centered at center
with axis of revolution directed by direction
and with radii major
and minor
.
Torus((0.,0.,0.), (1.,0.,0.), (0.,1.,0.), 0.2) |> viz
ParaboloidSurface
#
Meshes.ParaboloidSurface
— Type
ParaboloidSurface(apex, radius, focallength)
A paraboloid surface embedded in R³ and extending up to a distance radius
from its focal axis, which is aligned along the z direction and passes through apex
(the apex of the paraboloid). The equation of the paraboloid is the following:
where is the apex of the parabola, is the focal length, and is the clip radius.
ParaboloidSurface(apex, radius)
This creates a paraboloid surface with focal length equal to 1.
ParaboloidSurface(apex)
This creates a paraboloid surface with focal length equal to 1 and a rim with unit radius.
ParaboloidSurface()
Same as above, but here the apex is at Apex(0, 0, 0)
.
See also https://en.wikipedia.org/wiki/Paraboloid.
ParaboloidSurface((5., 2., 4.), 1.0, 0.25) |> viz